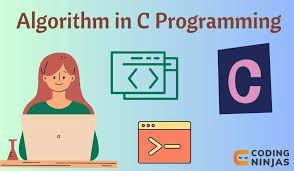
Course Title: Computer Programming Methodology with C Programming
Course Description:
This course introduces fundamental concepts in computer programming using the C programming language. It emphasizes problem-solving techniques, algorithm development, and structured programming methodologies. Students will learn to design, write, test, and debug programs, gaining a solid foundation in programming principles and best practices.
Course Objectives:
- Understand basic programming concepts, including data types, operators, control structures, and functions.
- Develop structured and modular programs using functions and file handling.
- Implement algorithms for problem-solving using loops, arrays, and pointers.
- Apply debugging techniques to identify and fix programming errors.
- Work with file operations and memory management in C.
- Foster good programming practices, including code readability and documentation.
Course Outline:
-
Introduction to Programming and C Language
- Overview of programming concepts
- Structure of a C program
- Compiling and executing C programs
-
Fundamentals of C Programming
- Variables, data types, and operators
- Input and output operations
- Conditional statements (if-else, switch)
-
Loops and Iterations
- While, do-while, and for loops
- Nested loops and break/continue statements
-
Functions and Modular Programming
- Defining and using functions
- Function prototypes, recursion, and scope
-
Arrays and Strings
- One-dimensional and multi-dimensional arrays
- String manipulation functions
-
Pointers and Memory Management
- Pointer basics and pointer arithmetic
- Dynamic memory allocation (malloc, calloc, free)
-
Structures and File Handling
- Structs and unions in C
- File operations (reading, writing, appending)
-
Debugging and Error Handling
- Common programming errors
- Debugging tools and techniques
-
Final Project and Assessment
- Implementation of a real-world application
- Code review and best practices
Prerequisites:
- Basic understanding of mathematics and logical thinking.
- No prior programming experience is required, but familiarity with computers is beneficial.
Assessment Criteria:
- Assignments and quizzes: 20%
- CAT: 40%
- Final Exam: 40%
- Attendance and participation: Mandatory
This course provides a strong foundation for further studies in computer science and software development, making it ideal for beginners and those looking to strengthen their programming skills.
- Teacher: IGIHOZO Didier